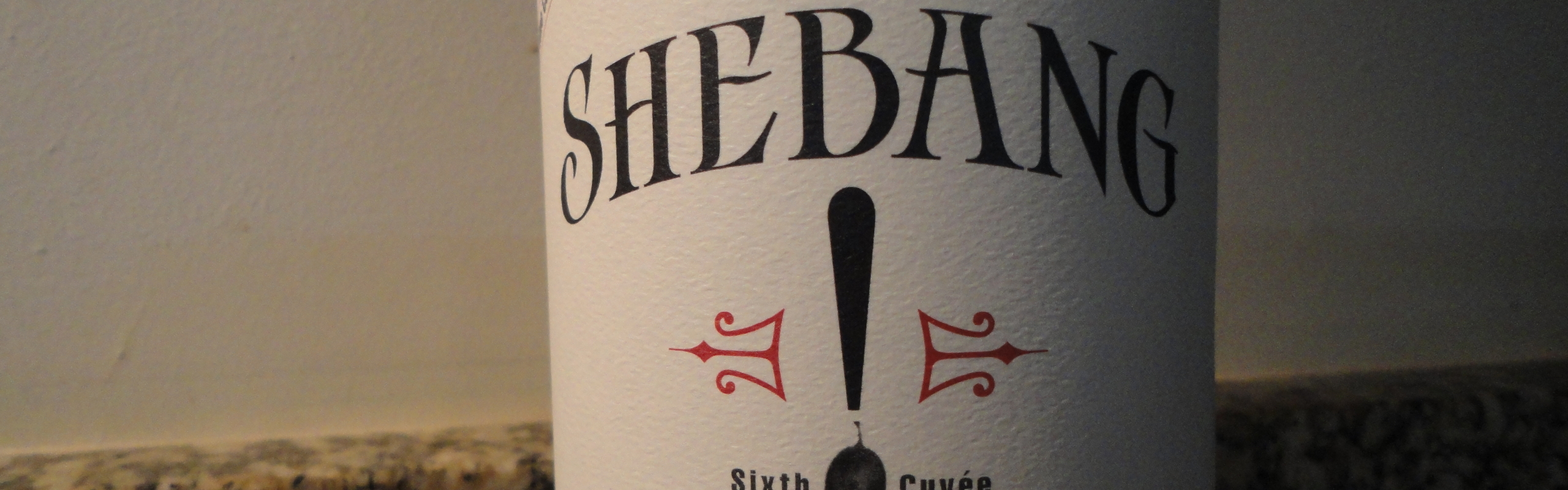
Hey folks, BASH is the core of most Linux system administrators' arsenal, and a good understanding of different techniques in BASH can be an absolute life-saver, so I've decided to compile and document a list of some of the interesting usages I've come across, both recently, and in my days at the office.
One liner conditional
One of the most useful quick and dirty command combinations I've come across is the one-liner conditional. This doesn't just mean using if condition; then do stuff; fi, as that's technically 3 lines, delimited with a semicolon. Here's an example:
[[ -e /sys/block/sda ]] && echo "Hard drive detected"
Now this could also have been achieved with a single square bracket, however I tend to use the double, as it has many more features. Here's an example:
[[ "Once upon a time" =~ ^Once*me ]] && echo "Pattern matches"
The double bracket can also do regex, as you can see. Here's a link to explain more.
Enter parameter if empty
This command will ask the user for input, should a variable not be set:
param=${param:-$(read -p "Enter parameter: "; echo "Parameter now set.")
This basically states that if the bash variable $param isn't set, set it to the value after the colon-dash, which in this case is a BASH subshell, which runs the read command. I find this commonly used in Slackbuild scripts (for compiling software for Slackware Linux)
Read a password in BASH (no character echo)
The read command can be used to read user input, but not echo it to the screen, such as during password entry. I recently used this to allow password entry in the following manner:
First, I created a password hash and stored it in a file as follows:
echo "MyPassword" | sha512sum > ~/password.sha
I then used the following code in a script to verify it:
#!/bin/bash
read -sp "Password: " PASSWORD
if ! [ "$PASSWORD" == "$(cat ~/password.sha)" ]; then
echo "Password entry failed."
exit 1
fi
echo "Password success! Continuing"
Take an X session screenshot from CLI
This is done using the xwd command, which will likely be preinstalled on most Linux distributions:
xwd -display localhost:0 -root > xsession.xwd
The file can then be converted into a more usable file using the convert command from the imagemagick package in the following way:
convert xsession.xwd xsession.png
Stop a command from going into BASH history
This one's quite well known, but I'm adding it so I remember it if I ever need it! The simple answer is to put a " " (space) before the command, like so:
# The original command
which bash
# The modified command
which bash
Change the BASH prompt
This can be incredibly useful. I have the following prompt on one of my file servers:
PS1="\n\[\033[35m\]\$(/bin/date)\n\[\033[32m\]\w\n\[\033[1;31m\]\u@\h: \[\033[1;34m\]\$(/usr/bin/tty | /bin/sed -e 's:/dev/::'): \[\033[1;36m\]\$(/bin/ls -1 | /usr/bin/wc -l | /bin/sed 's: ::g') files \[\033[1;33m\]\$(/bin/ls -lah | /bin/grep -m 1 total | /bin/sed 's/total //')b\[\033[0m\] -> \[\033[0m\]"
This shows the date in purple on the first line and the current working directory in green on the second. The third line contains a few snippets: The user and hostname, the current tty (pts/[0-9]), the number of files in the folder, and the size they take up. It looks like this (only with colour):
Tue Sep 27 21:16:32 BST 2011
~/Documents
n00b@server: pts/0: 120 files: 432Mb ->
I got this particular prompt from this site. For more information about colours in BASH scripts, try this site.
I reckon that's enough for today. I'll carry on collecting interesting snippets and posting them together in the future. Hope somebody out there found this useful!
n00b